I was recently doing some automation of a web procedure and ran into a problem that I had to go round and round to solve.
Initially I started by recording the interaction with the Selenium IDE and everything worked correctly in the test browser. The problem came when trying to move it to another browser and the web page required the browser to share the location.
By default all browsers block these requests and we have to give access individually to each domain. This is done with the classic modal request window like the following:
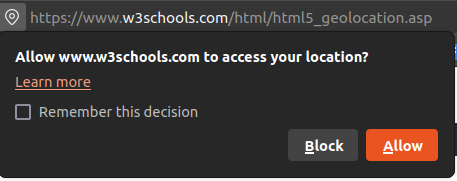
Once we have accepted it the browser will save that permission and the site will work correctly.

The problem is that when performing an automation with Selenium we don't necessarily start from a browser with a certain configuration. Therefore we will have to add this configuration in the tests so that it always works correctly.
The solution I am going to show is for Firefox and the tests are written in Python. In the creation of the webdriver we will have to pass a set of options that will become the preferences of the browser instance.
def setup_method(self, method):options = Options()options.set_preference('geo.enabled, True)options.set_preference('geo.prompt. testing.prompt.testing.allow.allow, True)options.set_preference('geo.prompt.testing.allow.allow, True)options.set_preference('permissions.default.geo.default.geo, True)options.set_preference('geo.provider.network. url, 'data:applicationJson,{"location: {"latelink: 40.7590, "lng: -73.9845}, "accuracy: 27000.0})self.driver = webdriver. Firefox(options=options)
In the JSON payload of the geo.provider.network.url field, we can modify the values of longitude, latitude and precision to the ones we are interested in for our case.
It is important to note that this method only works on recent versions of Firefox because the names of some preferences have changed.
Also note that this system is fully compatible with launching tests remotely in Selenium Grid.
Comments